간단한 입출력 기능을 가진 리액트 게시판을 하나 만들어보는 예제를 해보려고 한다.
나중에 소스코드도 올려둘테니 필요한 분들은 받아서 한번 살펴보길 바란다.
완성된 프로젝트 소스코드
github.com/esyeon88/simple-react-board
esyeon88/simple-react-board
simple type of board using react (ckeditor). Contribute to esyeon88/simple-react-board development by creating an account on GitHub.
github.com
소스코드 다운받아서 직접 해보기
2020/10/31 - [프로그래밍/backend] - [github] 프로젝트 소스코드 다운받아서 사용하기
[github] 프로젝트 소스코드 다운받아서 사용하기
맥북용으로 진행한다. 리눅스도 같은 방법으로 진행하면 된다. 윈도우는 추후에 따로 사용 방법을 올려 보도록 하겠다. 윈도우에서 리눅스 환경 구축하는 방법은 아래에서 확인할 수 있다. 2020/1
falaner.tistory.com
<사이트 예시>
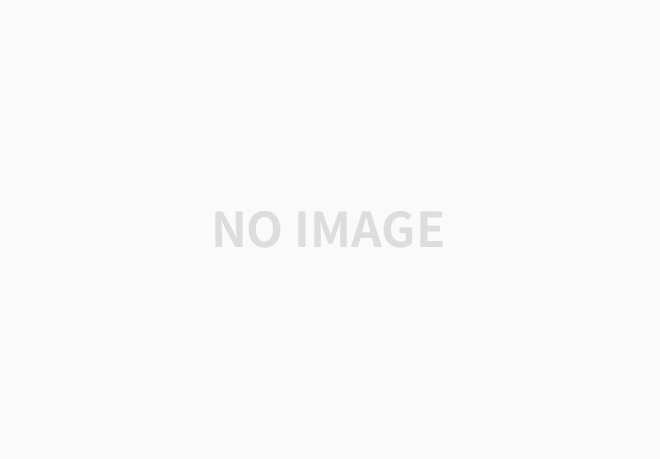
프로젝트 생성하기
먼저 리액트 프로젝트를 만들어 볼 폴더를 만들어준다.
원하는 위치에 다음 명령어를 입력하여 리액트 프로젝트를 생성한다.
simple-react-board 라는 프로젝트를 만든다.
$ npm create-react-app simple-react-board
그리고 디렉터리를 에디터화면에서 열어준다.
여기에서는 vscode 를 사용해서 진행한다.
폴더가 준비되었다면 다음 명령어를 입력하여 화면을 띄워본다.
예제에서는 yarn을 주로 사용하는데 npm을 사용하여 진행해도 상관없다.
$ yarn start
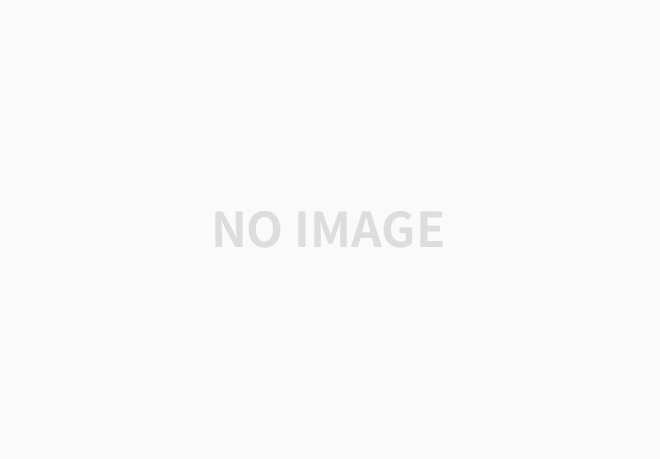
아래와 같은 화면을 볼 수 있다.
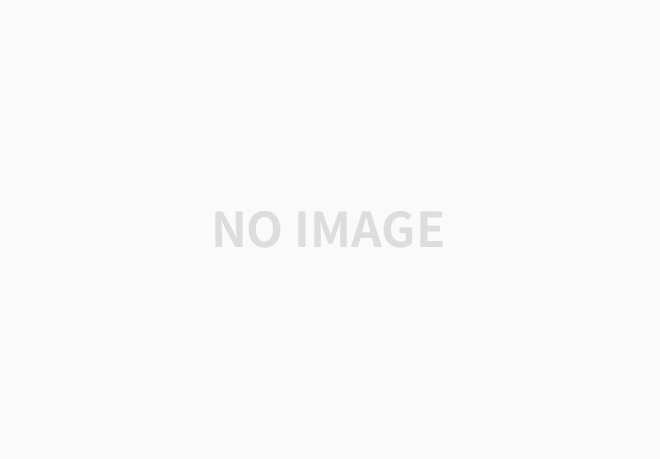
public 폴더 내에 로고파일들, 그리고 src 폴더안의 불필요한 파일들을 삭제해주고 app.js 파일을 열어 내용을 깨끗이 비워준다.
<index.js>
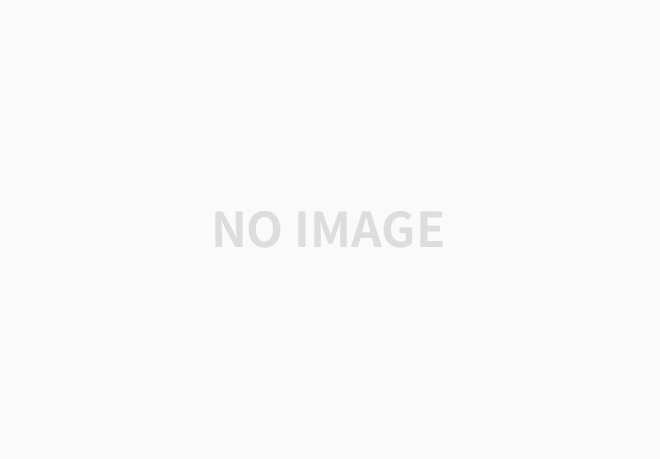
다음과 같이 수정
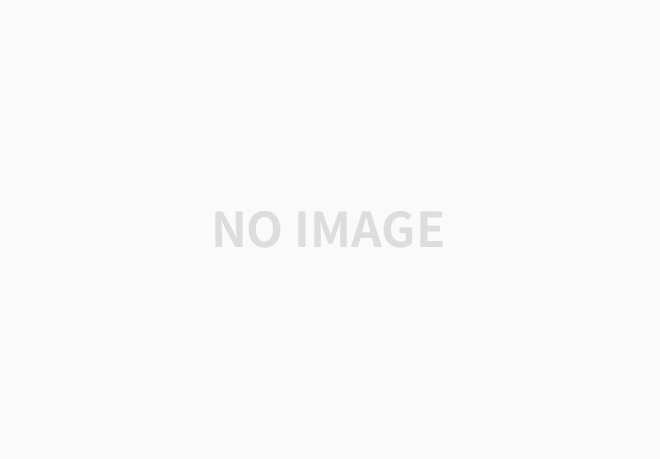
<app.js>
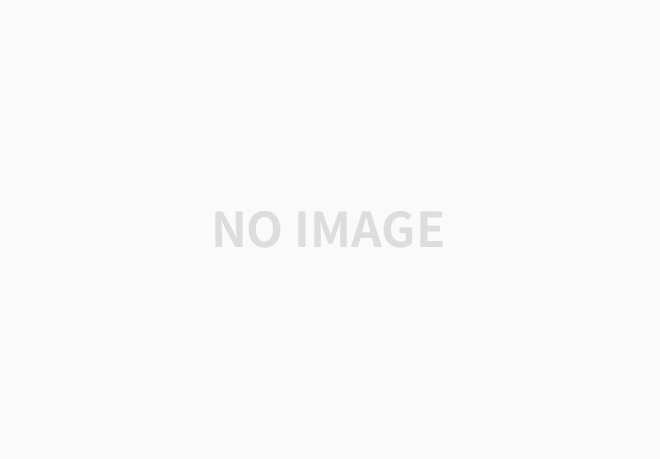
<브라우저 화면>
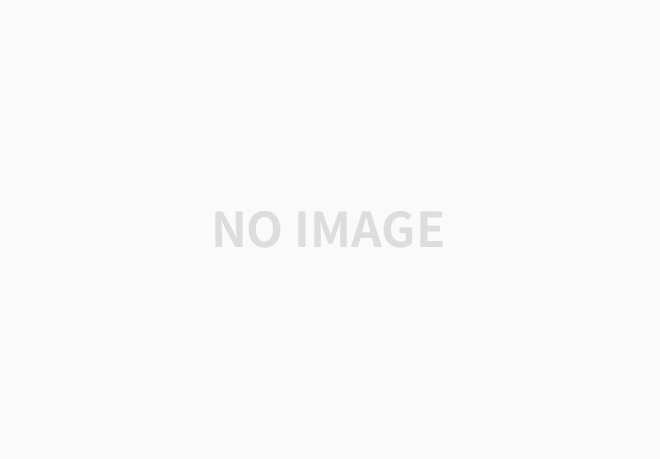
게시판 구조 만들기
대략 아래와 같은 구조의 사이트를 만들 계획이다.
제목과 내용을 입력하고 입력버튼을 누르면 위에 제목-내용 박스에 업로드 되는 형식이다.
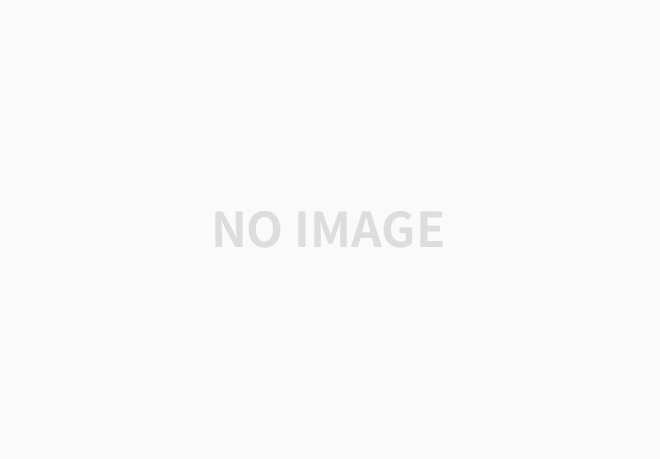
코드는 아래와 같은 구조로 만들 것이다.
<app.js>
import './App.css';
function App() {
return (
<div className="App">
<h1>Movie Review</h1>
<div className='movie-container'>
<h2>제목</h2>
<div>
내용
</div>
</div>
<div className='form-wrapper'>
<input className="title-input" type='text' placeholder='제목' />
<textarea className="text-area" placeholder='내용'></textarea>
</div>
<button className="submit-button">입력</button>
</div>
);
}
export default App;
<app.css>
.App {
text-align: center;
}
.movie-container {
margin: 0 auto;
width: 80%;
border: 1px solid #333;
padding: 10px 0 30px 0;
border-radius: 5px;
margin-bottom : 50px;
}
.form-wrapper{
width: 80%;
margin: 0 auto;
}
.title-input {
width: 500px;
height: 40px;
margin: 10px;
}
.text-area {
width: 80%;
min-height: 500px;
}
.submit-button {
width: 200px;
height: 50px;
font-size: 20px;
padding: 20px;
border: none;
background: indianred;
border-radius: 5px;
}
이제 위와 같은 구조에서 WYSIWYG 에디터인 ckeditor 를 설치해보자.
설치방법은 다음 포스팅에서 다뤘으니 따라서 해본다.
2020/10/27 - [프로그래밍/react] - [react]게시판 WYSIWYG(위지윅) 텍스트 에디터(ckEditor 5) 설치하기
작업폴더 터미널 내에서 다음 명령어를 입력하고 설치해준다.
$ npm install --save @ckeditor/ckeditor5-react @ckeditor/ckeditor5
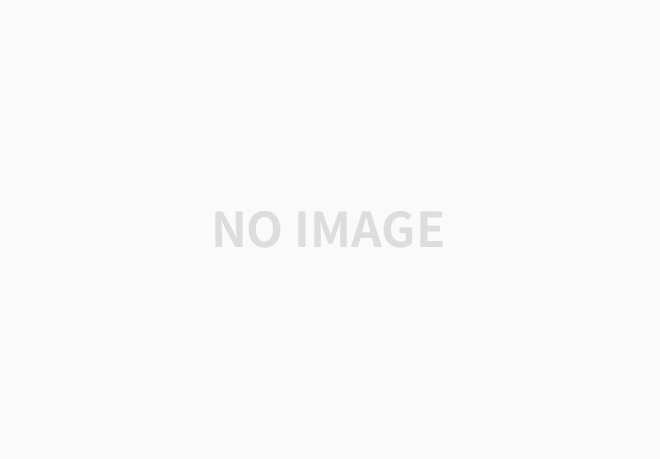
설치가 완료되었다면 코드를 다음과 같이 수정해준다.
<app.js>
import './App.css';
import { CKEditor } from '@ckeditor/ckeditor5-react';
import ClassicEditor from '@ckeditor/ckeditor5-build-classic';
function App() {
return (
<div className="App">
<h1>Movie Review</h1>
<div className='movie-container'>
<h2>제목</h2>
<div>
내용
</div>
</div>
<div className='form-wrapper'>
<input className="title-input" type='text' placeholder='제목' />
<CKEditor
editor={ClassicEditor}
data="<p>Hello from CKEditor 5!</p>"
onReady={editor => {
// You can store the "editor" and use when it is needed.
console.log('Editor is ready to use!', editor);
}}
onChange={(event, editor) => {
const data = editor.getData();
console.log({ event, editor, data });
}}
onBlur={(event, editor) => {
console.log('Blur.', editor);
}}
onFocus={(event, editor) => {
console.log('Focus.', editor);
}}
/>
</div>
<button className="submit-button">입력</button>
</div>
);
}
export default App;
그럼 다음과 같이 에디터가 설치된 모습을 확인할 수 있다.
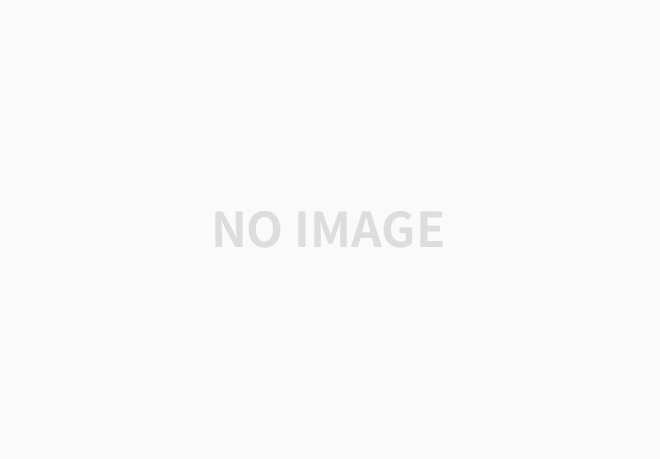
모양을 조금 예쁘게 다듬어보자.
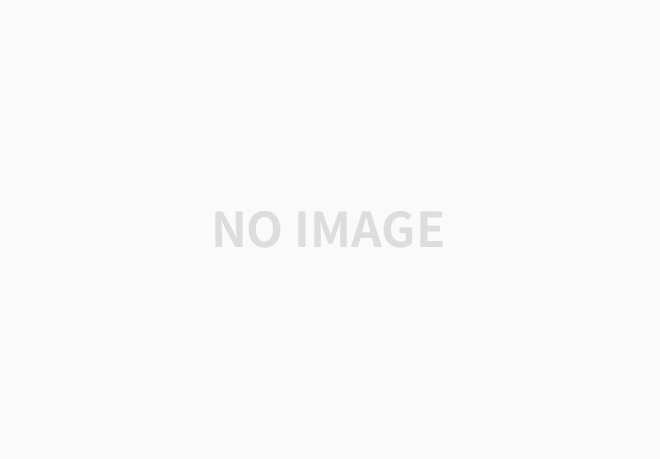
<app.css>
.App {
text-align: center;
}
.movie-container {
margin: 0 auto;
width: 80%;
border: 1px solid #333;
padding: 10px 0 30px 0;
border-radius: 5px;
margin-bottom : 50px;
}
.form-wrapper{
width: 60%;
margin: 0 auto;
}
.title-input {
width: 500px;
height: 40px;
margin: 10px;
}
.submit-button {
width: 200px;
height: 50px;
font-size: 20px;
padding: 20px;
border: none;
background: indianred;
border-radius: 5px;
margin-top: 40px;
vertical-align: middle;
}
.ck.ck-editor__editable:not(.ck-editor__nested-editable) {
min-height: 500px;
}
여기까지 게시판 인터페이스를 꾸며 보았다.
다음 포스팅에서는 내용을 입력하면 실제로 내용이 업데이트 되는 구조를 만들어 보려고 한다.
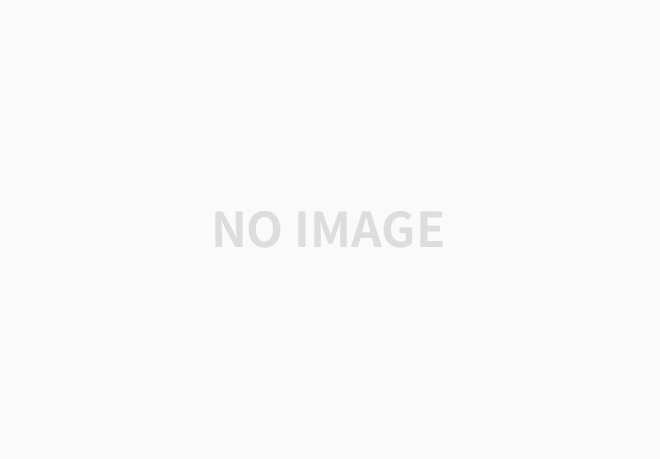